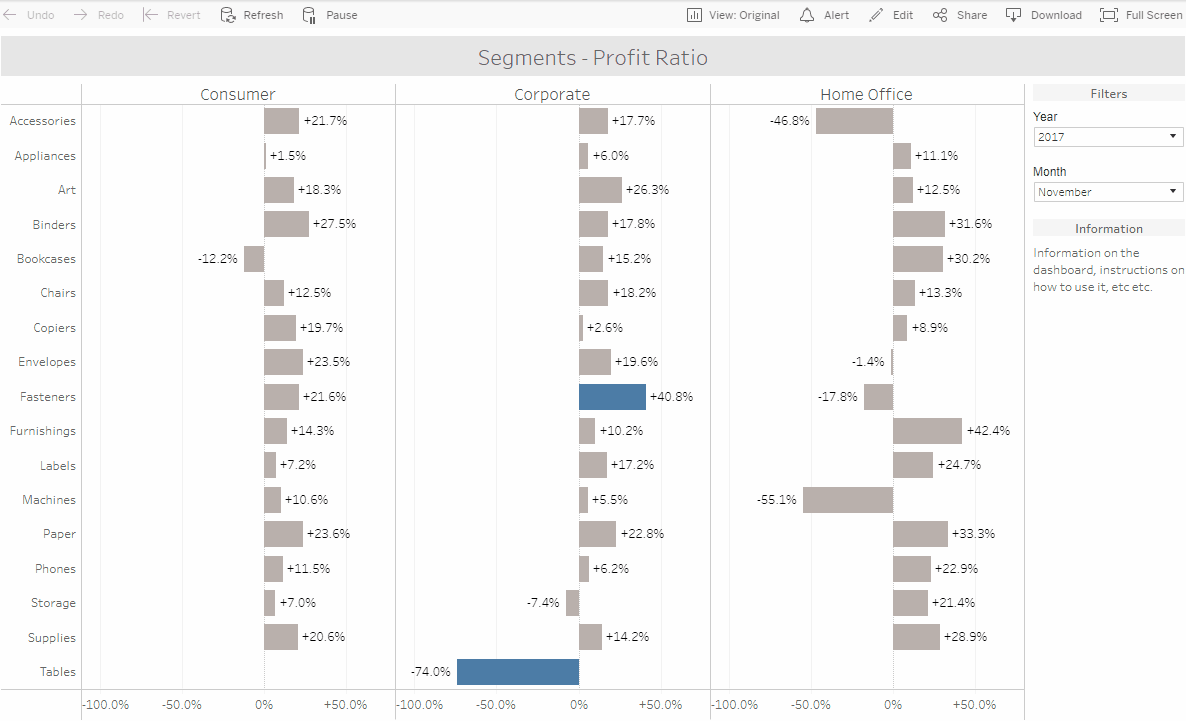
- The easiest method of commenting on dashboards, is to use Tableau Server's built-in commenting functionality. While no additional setup or configuration is required to use this method, it is also the least flexible method. Though it must be noted, the commenting functionality has been significantly improved in Tableau 10.4: http://onlinehelp.tableau.com/v10.4/pro/desktop/en-us/comment.html
- An alternative is to make use of a combination of Google Forms and Google Sheets. The form being filled out feeds the Google Sheet, which is then connected to the dashboard as a data source. The way that the form is integrated and filled out, can vary, but the form retains the look and feel defined by Google. Hence, this method requires slightly more preparation, but is somewhat more flexible.
- A third possibility, which will be explained in this post, is more complex in setup, but offers the highest level of flexibility. It consists of a custom web page, integrated into the dashboard, which feeds a database that will contain the comments (and feed these back).
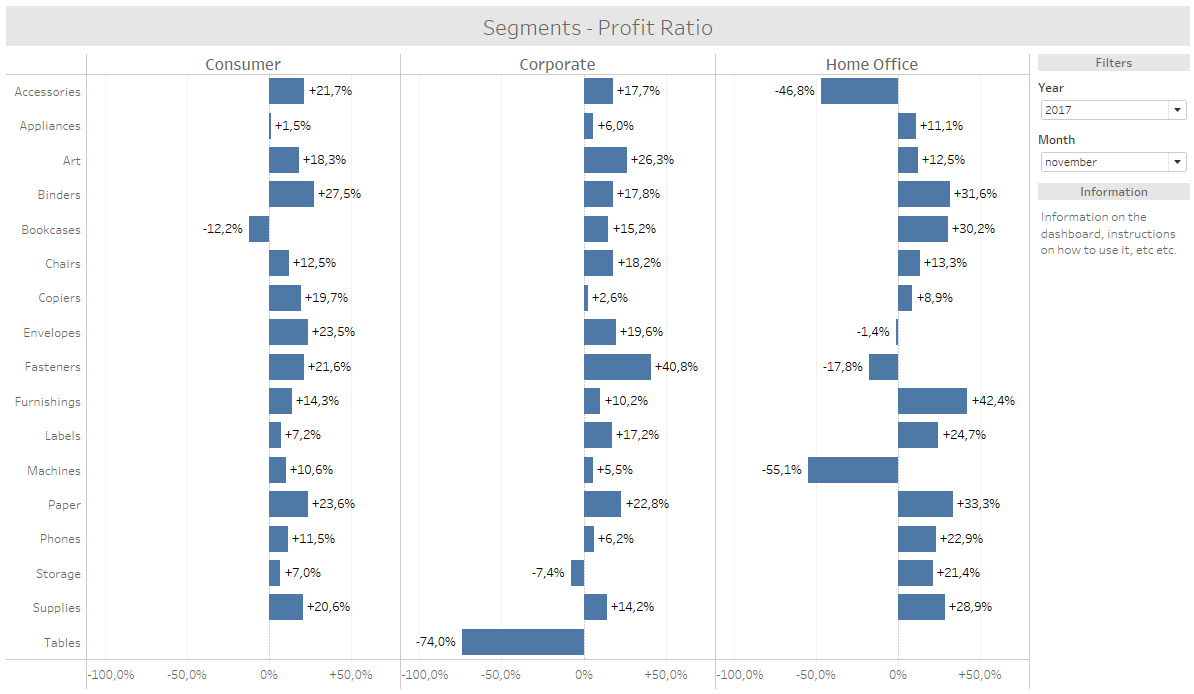
- Year
- Month
- Category
- Segment
- The comment itself.
- The Tableau username of the person who has posted the comment.
- Their full name.
- The timestamp of when the comment has been posted.
CREATE TABLE `comments_profitratio_segments` (
`category` varchar(50) NOT NULL,
`segment` varchar(50) NOT NULL,
`year` int(11) NOT NULL,
`month` int(11) NOT NULL,
`comment` varchar(1024) NOT NULL,
`username` varchar(255) NOT NULL,
`fullname` varchar(255) NOT NULL,
`insert_timestamp` timestamp NOT NULL DEFAULT URRENT_TIMESTAMP,
PRIMARY KEY (`category`,`segment`,`year`,`month`)
)
- The timestamp can be automatically filled in by how it is defined, although we will pass it from the web page sending the data.
- The primary key is a combination of the 4 dimensions we chose from our dashboard. In MySQL, this will allow us to "insert or update" comments in one query. That is, we'll be able to have MySQL decide if a certain comment already exists, and if so, update it.
- input_comment.php: actually more HTML than PHP, this is the page that displays the form for the entry of the comment by the user. This is the page that will be displayed when someone selects part of the chart, and clicks through on the link in the tooltip. We'll make sure it is displayed in the dashboard by using a Web Page object there.
- submit_insert.php: this is the PHP code which does the actual work: it will receive the comment (and necessary dimensions and metadata such as the username) from the first page, and insert the data into the table. It will then display either a message confirming the successful addition of the comment, or the error that may have been triggered.
input_comment.php:
<?php
include "head.html";
?><p>Category: <?php echo $_GET['category']; ?></p>
<p>Segment: <?php echo $_GET['segment']; ?></p>
<p>Period: <?php echo $_GET['year']; ?>, <?php echo $_GET['month']; ?></p>
<p>User: <?php echo $_GET['comment_username']; ?> (<?php echo $_GET['comment_fullname']; ?>)</p>
<form action="submit_insert.php" method="post">
Comment:<br>
<textarea name="comment" rows="15" cols="15">Enter your comment here.</textarea><br>
<input type="hidden" name="category" value="<?php echo $_GET['category']; ?>">
<input type="hidden" name="segment" value="<?php echo $_GET['segment']; ?>">
<input type="hidden" name="year" value="<?php echo $_GET['year']; ?>">
<input type="hidden" name="month" value="<?php echo $_GET['month']; ?>">
<input type="hidden" name="comment_username" value="<?php echo $_GET['comment_username']; ?>">
<input type="hidden" name="comment_fullname" value="<?php echo $_GET['comment_fullname']; ?>">
<input type="submit" value="Submit Comment">
</form>
<?php
include "foot.html";
?>
submit_insert.php:
<?php
include "head.html";ini_set('display_errors', 1);
$servername = "localhost";
$username = "comments";
$password = ""; // removed in this example
$dbname = "comments";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$category = $_POST['category'];
$segment = $_POST['segment'];
$year = $_POST['year'];
$month = $_POST['month'];
$comment = $_POST['comment'];
$comment_username = $_POST['comment_username'];
$comment_fullname = $_POST['comment_fullname'];
$category = $conn->escape_string($category);
$segment = $conn->escape_string($segment);
$year = $conn->escape_string($year);
$month = $conn->escape_string($month);
$comment = $conn->escape_string($comment);
$comment_username = $conn->escape_string($comment_username);
$comment_fullname = $conn->escape_string($comment_fullname);
echo "Category: $category<br>";
echo "Segment: $segment<br>";
echo "Year: $year<br>";
echo "Month: $month<br>";
echo "Comment: $comment<br>";
echo "Submitting comment as: $comment_username ($comment_fullname)<br>";
echo "<br>";
// A better statement that will replace when necessary (assuming the keys are correctly defined)
$sql = "INSERT INTO `comments_profitratio` (`category`, `segment`, `year`, `month`, `comment`, `username`, `fullname`, `insert_timestamp`) VALUES ('$category', '$segment', '$year', '$month', '$comment', '$comment_username', '$comment_fullname', now()) ON DUPLICATE KEY UPDATE `comment`='$comment', `username`='$comment_username', `fullname`='$comment_fullname', `insert_timestamp`=now()";
if ($conn->query($sql) === TRUE) {
echo "<p style='font-weight: bold'>Comment saved successfully.</p>";
} else {
echo "<p style='font-weight: bold; font-color: #990000'>Error: " . $sql . "<br>" . $conn->error . "</p>";
} $conn->close();
include "foot.html";
?>
- Add a Web Page object to the bottom right of the dashboard, leaving the URL blank for now.
- Go to Dashboard > Actions and create a new URL Action
- Name (for example, this can be anything): "Click here to add or modify a comment on <Segment>, <Category> (<MONTH(Order Date)> <YEAR(Order Date)>)"
- URL: the location of your web page, while passing the parameters for the applicable dimensions and filters. In my case:
http://ec2-54-212-228-31.us-west-2.compute.amazonaws.com/comments/input_comment.php?category=<Category>&segment=<Segment>&year=<FILTERVALUES(YEAR(Order Date))>&month=<FILTERVALUES(MONTH(Order Date))>&comment_username=<ATTR(Username)>&comment_fullname=<ATTR(Full Name)>
- Then what we can do as well, is change the settings for the tooltips to appear "on hover" instead of responsively; which I find slightly easier when using hyperlinks in comments.
- Then we test this setup by clicking one of the hyperlinks...
- Bingo! The web form, pre-populated with the correct values for our dimensions, appears on the right-hand side of the dashboard:
- We can then enter a comment and submit it as a test...
- And indeed! The comment has been inserted into the database:
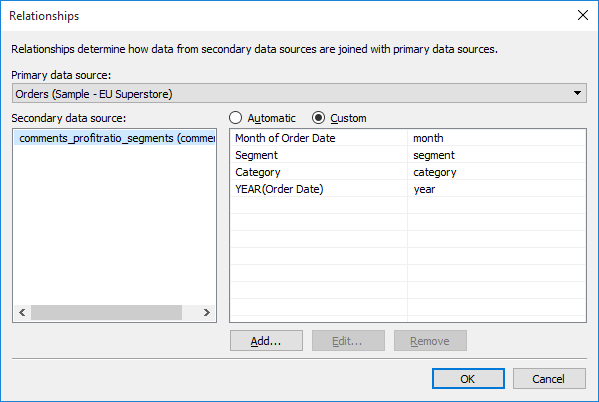
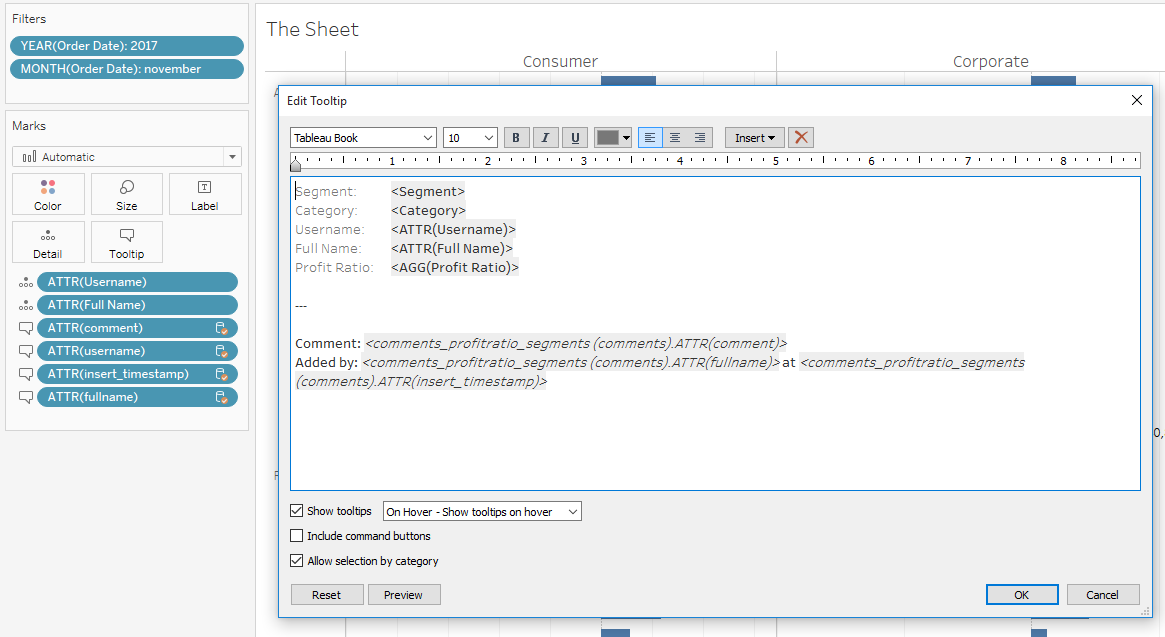
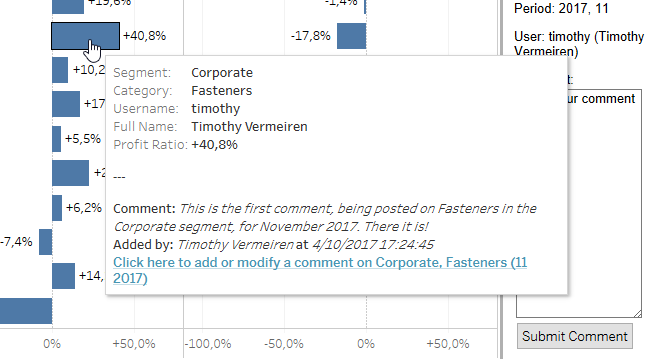
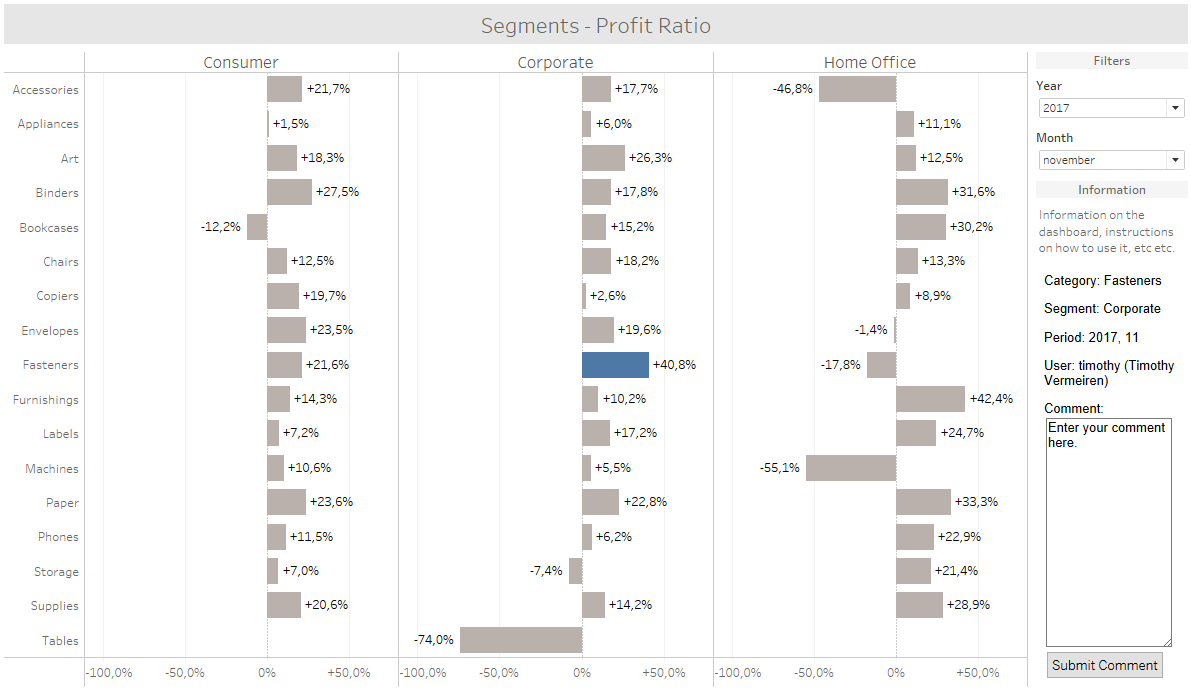